Context menus provide a simple way of displaying additional actions in response to a long-press on a View item. Some items in your view might require more options, but rather than cluttering a menu or the View with these options, they can be grouped together in a contextMenu.
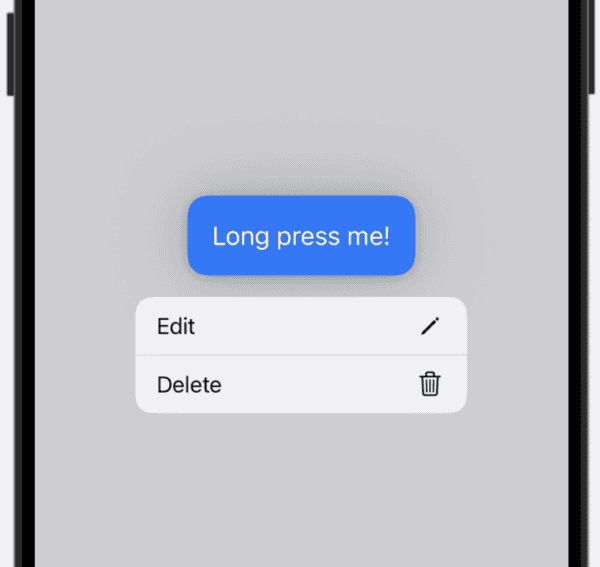
struct ContentView: View {
var body: some View {
VStack {
Text("Long press me!")
.padding()
.background(Color.blue)
.foregroundColor(.white)
.cornerRadius(8)
.contextMenu {
Button(action: {
print("Edit tapped")
}) {
Label("Edit", systemImage: "pencil")
}
Button(action: {
print("Delete tapped")
}) {
Label("Delete", systemImage: "trash")
}
}
}
.padding()
}
}
To create a contextMenu, you can use several types of Views to add the contextMenu to. In this example, it is added to a Text view.
Add the .contextMenu seen in line 9 of the code sample above, and then add in all of the buttons you need. Each button will be seen in the view in the order you add them to the .contextMenu. For the action, you can call a method or do whatever is needed.
Enhancing .contextMenu
The example above shows just two buttons that both look the same in regards to appearance. Lets modify things a little:
.contextMenu {
Button(action: {
print("Edit tapped")
}) {
Label("Edit", systemImage: "pencil")
}
Button(action: {
print("Move tapped")
}) {
Label("Move", systemImage: "folder")
}
Divider()
Button(role: .destructive, action: {
print("Delete tapped")
}) {
Label("Delete", systemImage: "trash")
}
}
Swap out the .contextMenu in the first example with the code sample above.
Another button has been added along with a Divider() and the last button has been set to the role of .destructive which makes the button red to provide a subtle warning to the user.
The divider just spaces the last button and Move button a little by introducing a small gap. This is useful if you have a long list of buttons in the contextMenu that could be divided by function or just to make it look more readable.
This is a relatively simple tutorial that gives your app a good way to handle cases where an item on the view needs to have more options, but putting them on the view permanently would be too distracting.
Leave a Reply
You must be logged in to post a comment.