If your user need a way to quickly copy something to the clipboard, or pasteboard as Apple calls it, in your iPhone app, then UIPasteboard is the class that can be used to accomplish this. UIPasteboard has been available since iOS 3.0.
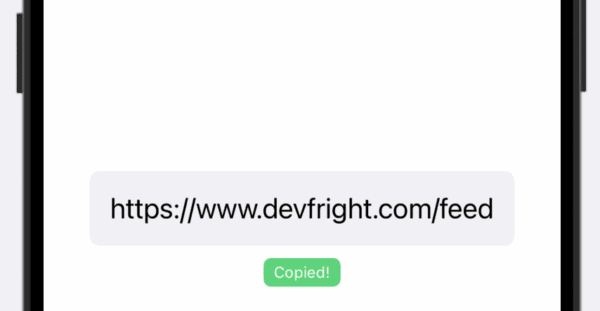
Implementing a Reusable View that uses UIPasteboard
We’ll look at creating a reusable View that uses the UIPasteboard that accepts a string and allows you to tap to copy.
struct CopyToPasteboardView: View {
@State private var showTooltip = false
let textToCopy: String
var body: some View {
VStack(spacing: 10) {
HStack {
Text(textToCopy)
.font(.title3)
.foregroundColor(.primary)
Image(systemName: "doc.on.clipboard")
.foregroundColor(.gray)
.imageScale(.small)
}
.padding()
.background(Color(.secondarySystemBackground))
.cornerRadius(8)
.onTapGesture {
UIPasteboard.general.string = textToCopy
withAnimation {
showTooltip = true
}
DispatchQueue.main.asyncAfter(deadline: .now() + 1.5) {
withAnimation {
showTooltip = false
}
}
}
if showTooltip {
Text("Copied!")
.font(.caption)
.padding(.horizontal, 8)
.padding(.vertical, 4)
.background(Color.green.opacity(0.8))
.foregroundColor(.white)
.cornerRadius(6)
.transition(.opacity.combined(with: .move(edge: .top)))
}
}
}
}
The view will work by accepting a string, and when that string is tapped, it will copy it to the pasteboard. A tool tip will show to let you know that the copy has happened.
Line 2 has a Bool called showToolTip that uses the @State property wrapper.
Line 3 has our textToCopy parameter that is required when the view is initialised.
We create our view with a VStack that has two Text views. The first is on line 8 that is styled and formats the text. This, and an Image view are wrapped in a HStack. The image used is from SF Symbols, see the SF Symbols tutorial here. This provides a prompt to the user to indicate that they can copy this text.
Lines 16 – 18 add some styling to the HStack.
On line 19 we use the .onTapGesture so that when the HStack is tapped, we store the textToCopy in the UIPasteboard.general.string property.
We add in withAnimation and inside that, on line 22, we set showToolTip to true. Because showToolTip uses @State, that Text view will animate and also push up the text to copy part of the view.
On line 25, on the main thread and after 1.5 seconds, we set showToolTip back to false and wrap that in withAnimation. This means that the tool tip shows for 1.5 seconds (animating in), and then disapears while animating out.
Using the CopyToPasteboardView
The final peice of the puzzle is making use of the CopyToPasteboardView. We use it as follows:
struct ContentView: View {
var body: some View {
CopyToPasteboardView(textToCopy: "https://www.devfright.com/feed")
.padding()
}
}
The ContentView is fairly self explanatory here. We make a call to our custom view called CopyToPasteBoardView and pass in the text we want to copy. For your app, that might be an email address, or anything you like.
One thing to note is that how this is setup now, you can copy just a single item. If you were to replace the string with an image, that image would overwrite the string, and vice-versa.
In another tutorial we’ll look at some more advanced features such as being able to store multiple items as well as different content types.
Leave a Reply
You must be logged in to post a comment.