I am currently in the process of building an email client app for iOS, and after that, the Mac. At the moment I am working on users being able to sign in to their Gmail account so that they can manage emails with within the app.
One of the first steps with working with the Gmail API is to be able to sign in. At the moment I have implemented GoogleSignIn which is one of a few ways that Google allows you to authenticate.
Creating a New Project
To begin, you can download this starter project which has a few things already set up, including Cocoapods. Please note that this part of the tutorial mostly follows what can be found on the Google Developers site.
A few notes on using Cocoapods:
- You need to have Cocoapods installed on your Mac. See install instructions here.
- You need to download the tutorial starter project from here.
- You need to navigate to the project in the terminal and run a command to install the dependencies. I already set up the podfile in the downloaded project for you, so you can skip that step. The command you run is: $ pod install
- When you open the sample app, you need to use the “Gmail.xcworkspace” file rather than the usual .xcodeproj file.
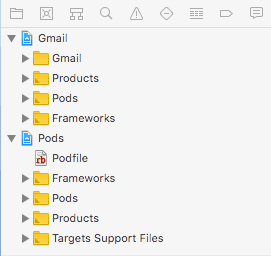
With the project opened you will see that the workspace has two projects inside. The Gmail app and one called Pods which is where Cocoapods downloads any specified dependencies to.
In the podfile you will see this line: pod ‘GoogleSignIn’ which I added. This instructs Cocoapods to install this dependency.
To access the dependency we need to open the ViewController.swift file found in Gmail > Gmail in the project navigator.
You will notice that we can now import GoogleSignIn as seen below.
import GoogleSignIn
You might notice that the class conforms to the GIDSignInUIDelegate protocol and that a number of methods are stubbed out.
class LoginViewController: UIViewController, GIDSignInUIDelegate {
The following delegate methods were added:
// MARK: - GIDSignInUIDelegate Delegates
func sign(inWillDispatch signIn: GIDSignIn!, error: Error!) {
//myActivityIndicator.stopAnimating()
}
func sign(_ signIn: GIDSignIn!, present viewController: UIViewController!) {
self.present(viewController, animated: true, completion: nil)
}
func sign(_ signIn: GIDSignIn!, dismiss viewController: UIViewController!) {
self.dismiss(animated: true, completion: nil)
}
The delegate methods above handle the sign in view that appears on screen when you tap a sign in button.
In viewDidLoad you will see:
GIDSignIn.sharedInstance().uiDelegate = self
This is used to set the delegate for the UI so that when the user taps the sign in button, for example, the sign in sheet is displayed.
In AppDelegate.swift you will notice that it also imports GoogleSignIn. It conforms to the GIDSignInDelegate (notice the slight difference between that and the GIDSignInUIDelegate found in the ViewController class).
In didFinishLaunchingWithOptions method we set the scopes (in this case, the whole of Gmail, although that is far too much for what this tutorial needs), and then set the clientID (provided by Google), set the scopes, set the delegate to self, and signInSilently.
Towards the bottom of the class we also implement two delegate methods (didSignInFor, and didDisconnectWith).
Back to the clientID, you will need to follow the instructions found here to get a client ID as well as get the other items you need to fully integrate GoogleSignIn.
I have left a template in of the .plist file for credentials, although you will need to populate it with your own data. I have also created the URL scheme, which you will also need to edit with your data.
In the Storyboard you will notice I dragged out an empty UIView and changed its class to be of type GIDSignInButton. You can connect it up to an IBOutlet if you want to adjust how it looks. I decided not to in this example and just leave the default Google Sign in button visible.
I added a couple of UILabels to the Storyboard that are populated with the name and email address of the account that you logged in to. I didn’t delve too much in to this part, so once the signup is complete and you are logged in, you’ll need to hit the signup button again to populate those labels.
Any questions, then please comment below and I’ll do my best to answer you.
Leave a Reply
You must be logged in to post a comment.