In the last tutorial, we looked at ChatGPT chat conversations and added a simple user interface to a SwiftUI app. Today we will look at integrating DALL-E into your SwiftUI app, using the image generations endpoint that allows you to provide a prompt and generate one to ten images. The app will be similar to what was created yesterday. The difference is that instead of receiving an answer in text form, we’ll receive it as a URL that can then be processed to display on the view.
[Read more…]ChatGPT Integration: Building a SwiftUI Chatbot for Your iPhone App
In the past year, ChatGPT has brought AI to the limelight. ChatGPT 3 was the model in use when I first heard of it last year. Just like it did with me, It has captured the interest of many people and businesses.
ChatGPT can be used for many things, such as finding answers to daily questions, solving maths problems, writing blog posts, and writing code, to name a few.
In this tutorial, we’ll connect a SwiftUI app to OpenAI’s ChatGPT API to provide a chatbot for your mobile app. The tutorial will briefly cover how to create a simple user interface and connect to OpenAI’s API to receive answers to questions you ask within the app.
The application here is just a chatbot, but you can use what you learn here to add value to any kind of app such as a note-taking app where a user can write some notes and then use AI to either add, summarise or correct what has been written.
OpenAI’s API is very similar to many other APIs on the internet in that you create an HTTP request, such as a POST request, add the bearer to the header, add items to the body, and then post it. The API then passes back a result that contains the answer you need.
[Read more…]Learning C# and .NET
I started DevFright in 2013. I was new to programming at that time. I had done a class in the mid-90s learning Pascal, but that only scratched the surface. I dabbled a bit with PHP on some WordPress installs that I had, but it wasn’t until 2012 that I started being more serious about programming. I began with iTunes-U content from Stanford, and in 2013 I started writing about what I was learning.
I created DevFright to write about what I was learning about creating iPhone apps. I had been learning Objective-C and felt I could share my learning.
Most of my work until late 2019 was with Objective-C and Swift. That year, I became more involved with PHP, using a custom framework to create an API. Shortly after that, I worked in Lumen and then switched to Laravel.
Things changed again when I began working in Python with a few friends so that we could learn the language together. We built the beginnings of a chess game and tried to engineer it so that the classes and relationships between them made sense. It was a great few months spending a few hours together each week.
In 2021 I began moving more to using Python with some JS and TS as well. I helped build an API that used Python and FastAPI. The team changed, and suddenly we discussed building something more reliable, perhaps using native code to create a separate iPhone and Android app rather than a PWA that was causing grief.
We decided to switch to C# and .NET using MAUI, which was still in beta then.
Surprisingly to me, I like C#. It has been great to work with. I am still very new at using it
[Read more…]Learning SwiftUI
I have written a handful of tutorials about SwiftUI, but since it was announced in 2019, I’ve done very little with it. At the end of 2019, I stepped away from iOS dev work and, for several months, worked with PHP, a custom framework, and the Laravel and Lumen frameworks to do some backend work.
In late 2020, I took a break due to an injury. In 2021, I did some work in JavaScript, VueJS, and Python and then moved on to C# in 2022. Mobile dev is where I prefer to work. Although I do some mobile dev work in .NET MAUI, it isn’t quite the same experience as a full native experience, in my opinion, although MAUI is good. I might write about that over on my dev site.
[Read more…]How to add a Map to a SwiftUI App
Many iPhone apps use maps to display helpful information to the user, often showing the current location. Uses include maps for navigation, finding nearby places, adding location to notes, reminders, and so on. This short tutorial will teach us two ways to add a map to an app.
Using MapKit
The MapKit framework makes adding a map to your app simple. You can first begin by importing MapKit into your view as follows:
import MapKit
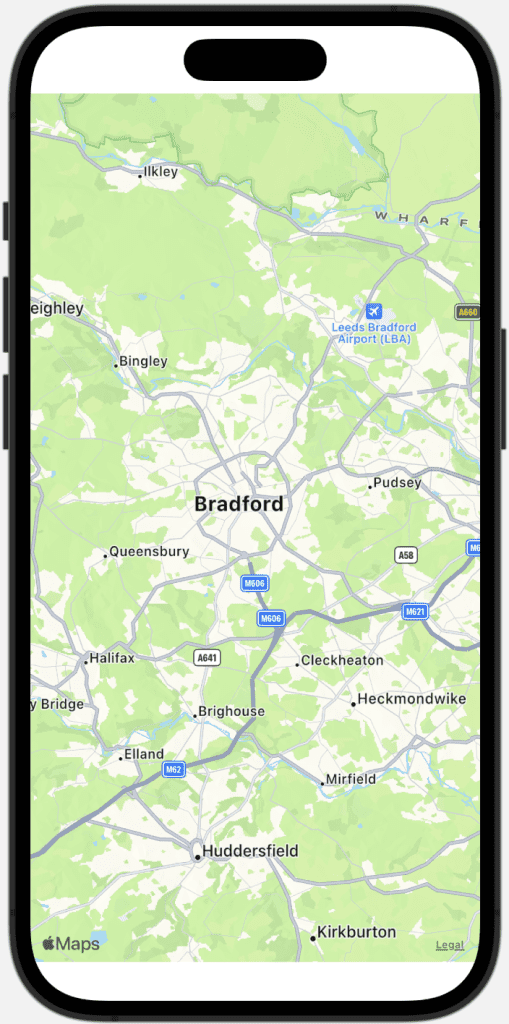
How to Use Local Notifications
There are two categories of notifications on iOS devices. One type is a push notification sent by a server, and the other is a local notification scheduled on-device that appears at a particular time or location.
This tutorial focuses on local notifications. An example of a local notification can be seen in your calendar. You add an appointment, set it to remind you 15 minutes before, and 15 minutes before the meeting begins, you see a notification on your lock screen. Let’s take a look at how these work.
[Read more…]The SwiftUI App Life Cycle
When Xcode 12 was launched in 2020 it brought with it a new app life cycle for SwiftUI applications. Prior to this, SwiftUI based apps were using AppDelegate.swift and SceneDelegate.swift.
Creating a new app can be done with this code:
import SwiftUI
@main
struct MyNewApp: App {
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
Apple Sample Code for Three-Column Layout in SwiftUI
For the last few weeks, I have been making plans for a new iPad/iPhone, and potentially macOS application. I haven’t used SwiftUI much in development so wasn’t sure how best to sort out the navigation on both mobile devices.
The app I am building, which is in very early stages, is for note-taking and meeting planning. My plan was to use a two-column layout, much like master/detail, but it seems that one good option is the three-column layout that has a menu on the far left column, a list view in the centre column, and then details on the rest of the view, as seen below.
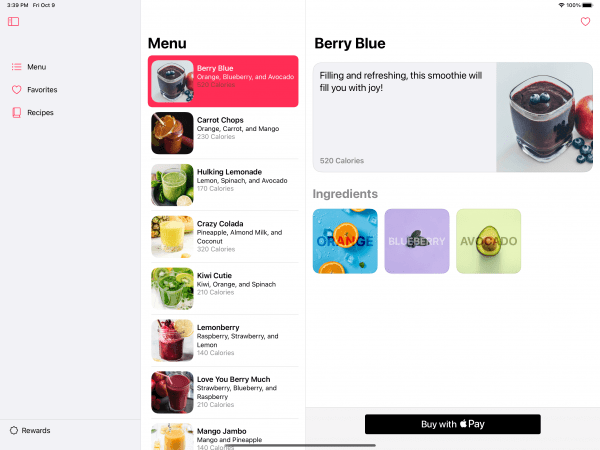
Unable to use SwiftUI Preview due to Insufficient System Resources
If you are getting an error saying that you cannot preview this file (while using SwiftUI), then perhaps like my experience, a reboot might fix it. That’s the short version to possibly get you going before reading on.
I created a new project today and switched the default ContentView.swift to use an iPad Pro 11 inch for the preview. An error appeared in right pane saying “Cannot preview in this file – Unable to boot device due to insufficient system resources”. Clicking the diagnostics button provided the following:
HumanReadableNSError: Unable to boot device due to insufficient system resources.
Please see Simulator Help for information on adjusting resource limits.
NSPOSIXErrorDomain (67):
==NSLocalizedFailureReason: The current system settings are not sufficient to allow booting additional simulators: maxFiles: 12288, openFiles: 11669, enforcedFilesBuffer: 1868
As mentioned, a reboot fixed the issue. It seems that Apple last recognised this happening back in 2017 in the Simulator Help section on the Apple help site that gives some recommendations on how to modify the number of processes and number of open files.
What is a Swift Class
We recently wrote about Swift structs. In this tutorial, we’ll look at classes. Classes and structures have a lot in common, although there are some important differences. Let’s take a look at what a class is and what you use them for.
- A class is initialised as an object.
- You need to create an initialiser for your class.
- Classes can also inherit from other classes which bring to your class the abilities and properties of the other class. You can also override the default behaviour of a method of the parent class.
- Unlike structs which are passed around by value, classes are passed by reference.
- « Previous Page
- 1
- 2
- 3
- 4
- …
- 13
- Next Page »